Since: Aug 2018
Licence: MIT
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
1.3. Verifying the setup
-
Run the
seedu.address.MainApp
and try a few commands. To find this particular file, refer to Figure 1 for guidance.

-
Run the tests to ensure they all pass. Refer to Figure 2.
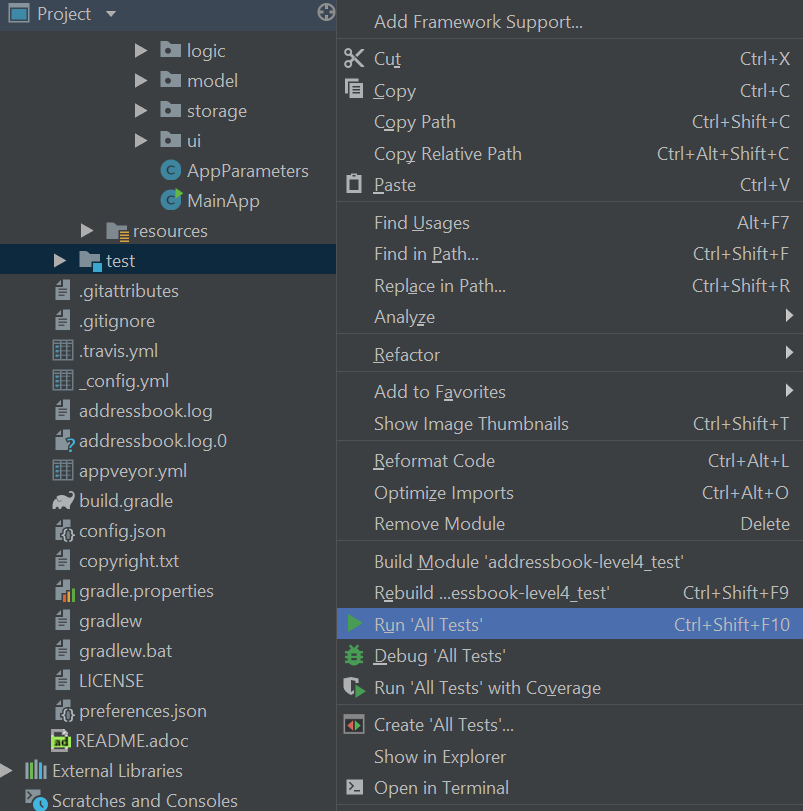
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still have the SE-EDU branding and refer to the se-edu/addressbook-level4
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to se-edu/addressbook-level4
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at Appendix A, Product Scope.
2. Design
2.1. Architecture
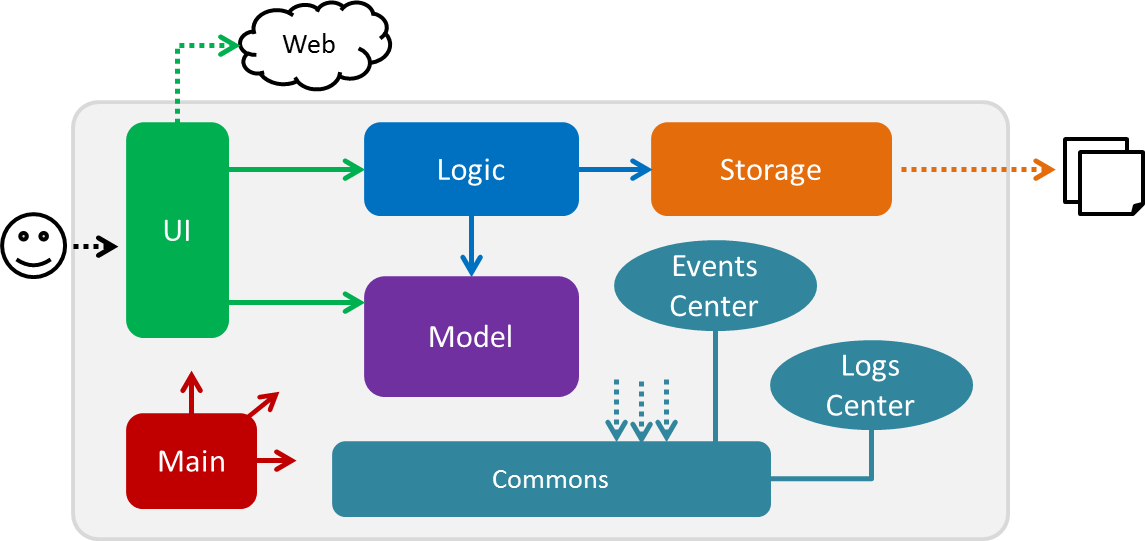
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
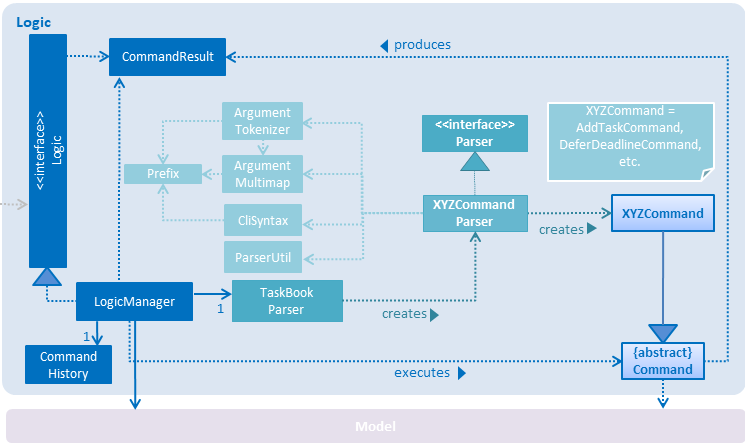
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command delete 1
.
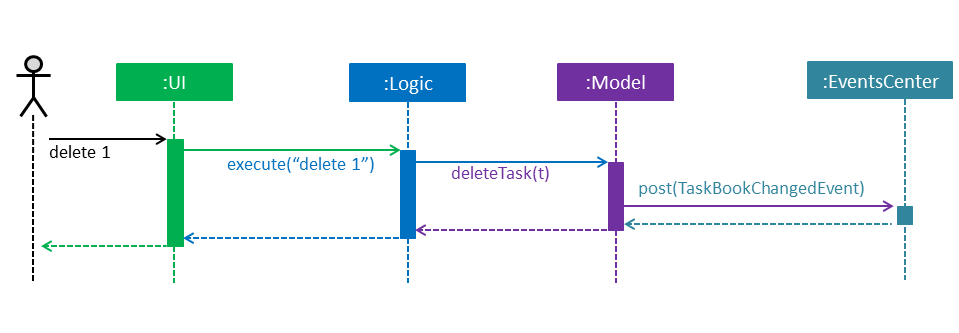
delete 1
command (part 1)
Note how the Model simply raises a AddressBookChangedEvent when the Address Book data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
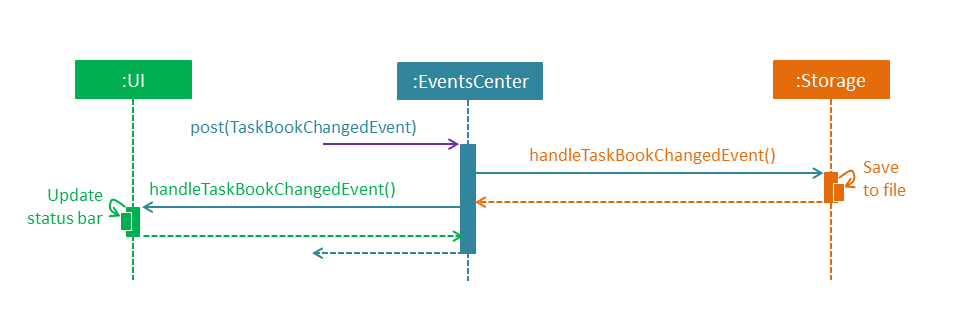
delete 1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
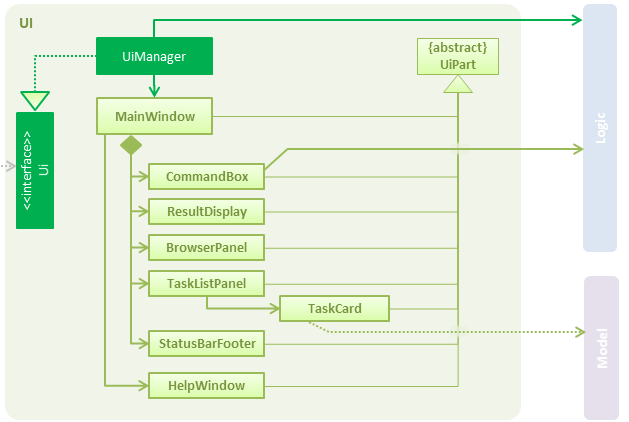
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
, TaskListPanel
, StatusBarFooter
, BrowserPanel
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
2.3. Logic component
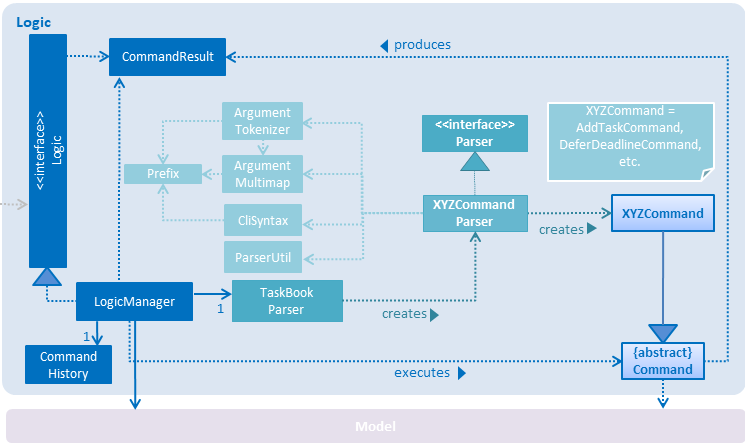
API :
Logic.java
-
Logic
uses theTaskBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a task) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
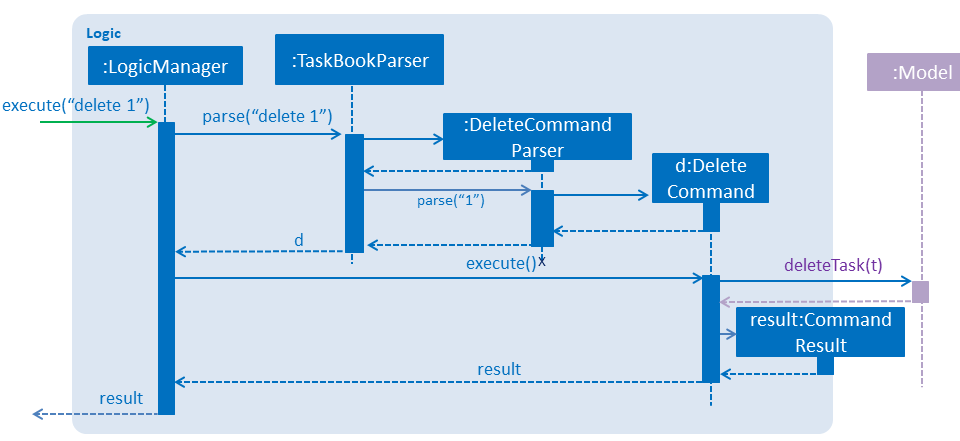
delete 1
Command2.4. Model component
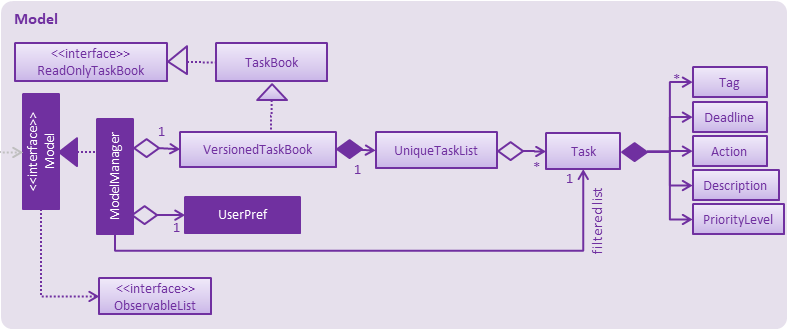
API : Model.java
The Model
,
-
stores a
UserPref
object that represents the user’s preferences. -
stores the Task Book data.
-
exposes an unmodifiable
ObservableList<Task>
that can be 'observed' e.g. the UI can be bound to this list so that the UI automatically updates when the data in the list change. -
does not depend on any of the other three components.
2.5. Storage component
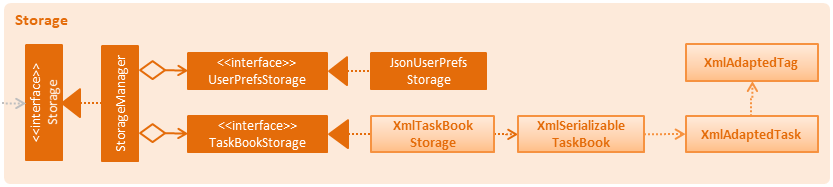
API : Storage.java
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Task Book data in xml format and read it back.
2.6. Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. Select Deadline feature
3.1.1. Current Implementation
The select
mechanism is facilitated by VersionedTaskBook
.
-
VersionedTaskBook#selectDeadline()
- Selects date to be set as deadline -
indicateTaskBookChanged()
— Event raised to indicate that the TaskBook in the model has changed.
These operations are exposed in the Model
interface as Model#selectDeadline()
, Model#updateFilteredTaskList()
and Model#commitTaskBook()
respectively.
Given below is an example usage scenario and how the select
mechanism behaves are each step.
Step 1. The user launches the application. If it is the first time he/she is launching it, the VersionedTaskBook
will be initialized with a sample task book data. If the user has already launched it previously and made changes to it, the VersionedTaskBook
launched will contain the data that he/she has entered in the previous launch.
Step 2. The user executes select 1/1/2018
or select dd/1 mm/1 yyyy/2018
to select the deadline.
Step 3. The select
command will call Model#selectDeadline()
and select the deadline as 1/1/2018. Model#updateFilteredTaskList()
will be called within Model#selectDeadline()
to update the list that is being shown to the user with only show tasks with 1/1/2018 as the deadline.
Step 4. Lastly, Model#commitTaskBook()
will be called to update the TaskBookStateList
and currentStatePointer
.
The following sequence diagram illustrates how the select
command is implemented.
select
command3.1.2. Design considerations
Aspect: Format for selection of date
-
Alternative 1 (current choice): Allows users to use string dd/mm/yyyy format
-
Pros: Easy for users to type command as it is more intuitive without the need to type in all the prefixes.
-
Cons: A separate method of parsing has to be created to handle such a format.
-
-
Alternative 2: Only allow format with prefixes.
-
Pros: No need for a separate method to handle another format by limiting users to only the format with prefixes.
-
Cons: Users may find it difficult to enter the date since it is less intuitive which might result in multiple failed attempts to do so.
-
Aspect: Method to set deadline
-
Alternative 1 (current choice): Deadline is selected by a separate
Select
deadline function.-
Pros: Allows users to add multiple tasks to the same deadline without having to type the deadline over and over again. Also allows a
filteredTaskList
based on deadline to be shown to users for them to see how many tasks are currently added to the current deadline. -
Cons: A separate function has to be created to select the date.
-
-
Alternative 2: Deadline is selected as a field in the
add
command.-
Pros: Separate
select
function is not required since deadline is set with everyadd
command. -
Cons: Users have to type the deadline repeatedly if multiple tasks are added to the same deadline. A separate function has to be created to view only tasks that are added to a specific deadline.
-
3.2. Edit Task feature
3.2.1. Current Implementation
The edit
mechanism is facilitated by VersionedTaskBook
.
Additionally, it implements the following operations:
-
Model#getFilteredTaskList()
— Obtains the current list of Tasks that is being displayed to the user -
VersionedTaskBook#updateTask()
— Edits and updates the specified task with the new values. -
indicateTaskBookChanged()
— Event raised to indicate that the TaskBook in the model has changed.
These operations are exposed in the Model
interface as Model#updateTask()
, Model#updateFilteredTaskList()
and Model#commitTaskBook()
respectively.
Given below is an example usage scenario and how the edit
mechanism behaves at each step.
Step 1. The user launches the application. If it is the first time he/she is launching it, the VersionedTaskBook
will be initialized with a sample task book data. If the user has already launched it previously and made changes to it, the VersionedTaskBook
launched will contain the data that he/she has entered in the previous launch.
Step 2. The user executes edit i/1 t/Complete CS2113 tutorial
to edit the title of the existing task at index 1 in the list. The edit
command obtains the data of the task that the user wants to change based on the input index.
Step 3. The edit
command will retrieve and copy the details of the task at index 1 to a new task editedTask
and edit the title to Complete CS2113 tutorial
.
Step 4. The edit
command will call Model#updateTask()
and update the task at index 1 with the details of editedTask
. Also, Model#updateFilteredTaskList()
will be called to update the list that is being shown to the user with the updated task.
Step 5. Lastly, Model#commitTaskBook()
will be called to update the TaskBookStateList
and currentStatePointer
.
The following sequence diagram illustrates how the edit
command is implemented.
edit
command3.2.2. Design considerations
Aspect: How to edit different fields of a task
-
Alternative 1 (current choice): Allows users to specify which fields they want to change using
Prefix
-
Pros: Users can change more than one fields at a time.
-
Cons: Command might be longer and harder for users to type.
-
-
Alternative 2: Have individual functions for editing every field
-
Pros: Easy to implement.
-
Cons: Too many different commands for the users to handle.
-
Aspect: Choosing a task to edit
-
Alternative 1 (current choice): Choose a
Task
based onIndex
from thefilteredTaskList
.-
Pros: Easy to implement since Index is already implemented in TaskBook.
-
Cons: Users have to scroll through the entire list of tasks to find the
Task
they want to edit. If the currentfilteredTaskList
shown to them does not contain the task they want to edit, they have to use the ‘List’ command first, resulting in a even longer list of tasks to filter.
-
-
Alternative 2: Choose a
Task
by a find command-
Pros: Users do not have to scroll through long task lists to find the task they want to edit.
-
Cons: A separate find command has to be implemented. Since there may be task titles that are similar or even the same, the find command will have to show a
filteredTaskList
for users to select the task to be edited by index which is similar to the first implementation.
-
3.3. Undo/Redo feature
3.3.1. Current Implementation
The undo/redo mechanism is facilitated by VersionedTaskBook
.
It extends TaskBook
with an undo/redo history, stored internally as an taskBookStateList
and currentStatePointer
.
Additionally, it implements the following operations:
-
VersionedTaskBook#commit()
— Saves the current task book state in its history. -
VersionedTaskBook#undo()
— Restores the previous task book state from its history. -
VersionedTaskBook#redo()
— Restores a previously undone task book state from its history.
These operations are exposed in the Model
interface as Model#commitTaskBook()
, Model#undoTaskBook()
and Model#redoTaskBook()
respectively.
Given below is an example usage scenario and how the undo/redo mechanism behaves at each step.
Step 1. The user launches the application for the first time. The VersionedTaskBook
will be initialized with the initial task book state, and the currentStatePointer
pointing to that single task book state.

Step 2. The user executes delete 5
command to delete the 5th task in the task book. The delete
command calls Model#commitTaskBook()
, causing the modified state of the task book after the delete 5
command executes to be saved in the taskBookStateList
, and the currentStatePointer
is shifted to the newly inserted task book state.
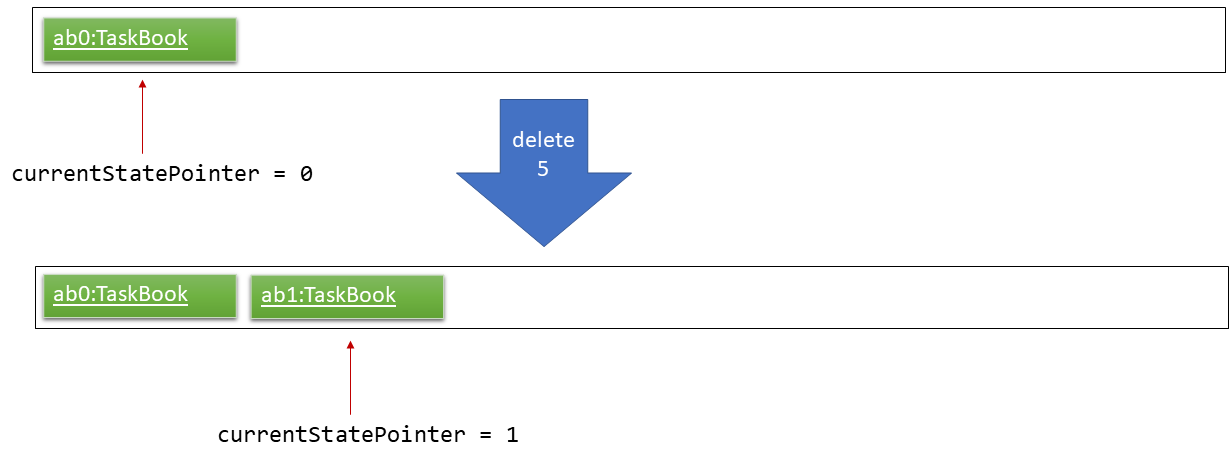
Step 3. The user executes add t/Do math homework …
to add a new task. The add
command also calls Model#commitTaskBook()
, causing another modified task book state to be saved into the taskBookStateList
.
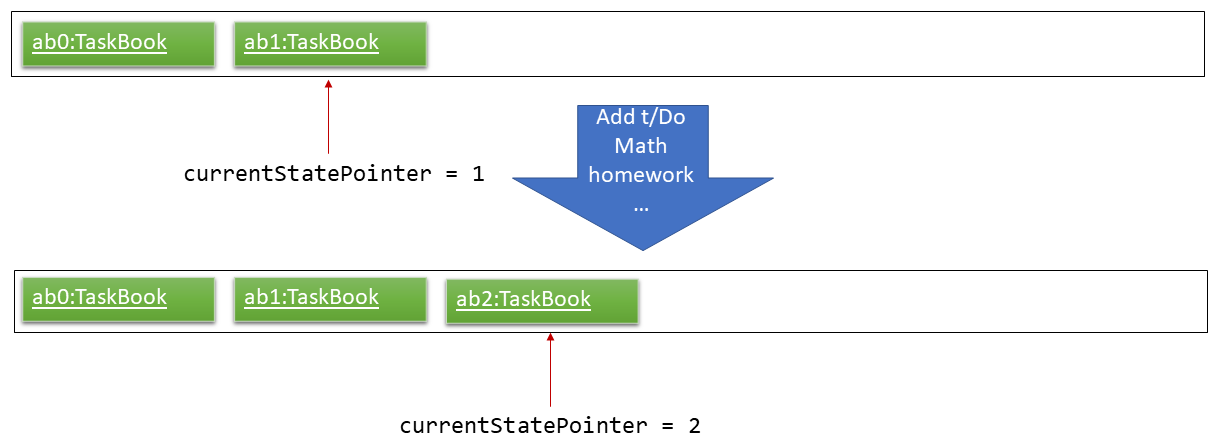
If a command fails its execution, it will not call Model#commitTaskBook() , so the task book state will not be saved into the taskBookStateList .
|
Step 4. The user now decides that adding the task was a mistake, and decides to undo that action by executing the undo
command. The undo
command will call Model#undoAddressBook()
, which will shift the currentStatePointer
once to the left, pointing it to the previous task book state, and restores the task book to that state.
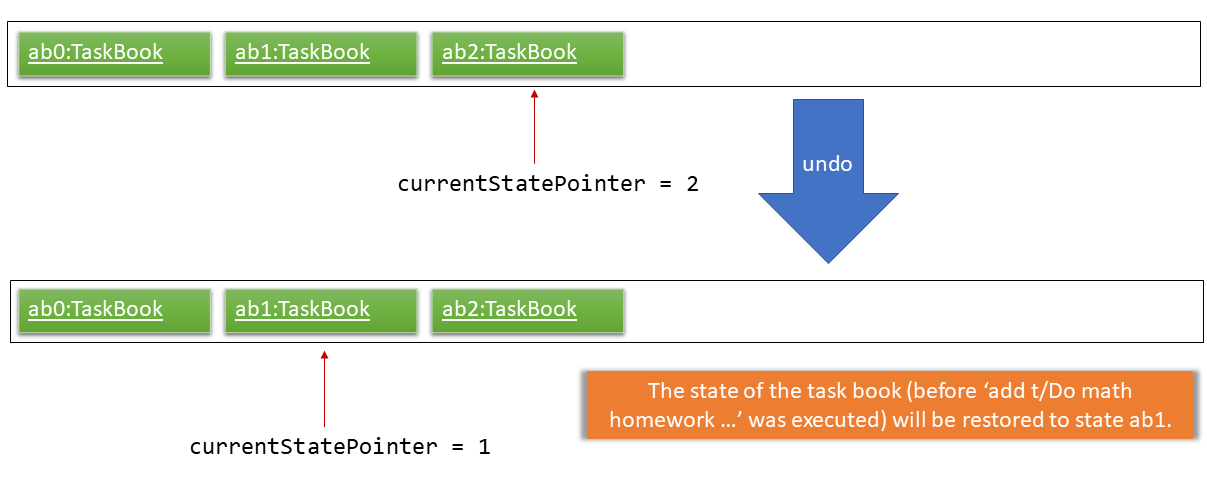
If the currentStatePointer is at index 0, pointing to the initial task book state, then there are no previous task book states to restore. The undo command uses Model#canUndoAddressBook() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the undo.
|
The following sequence diagram shows how the undo operation works:
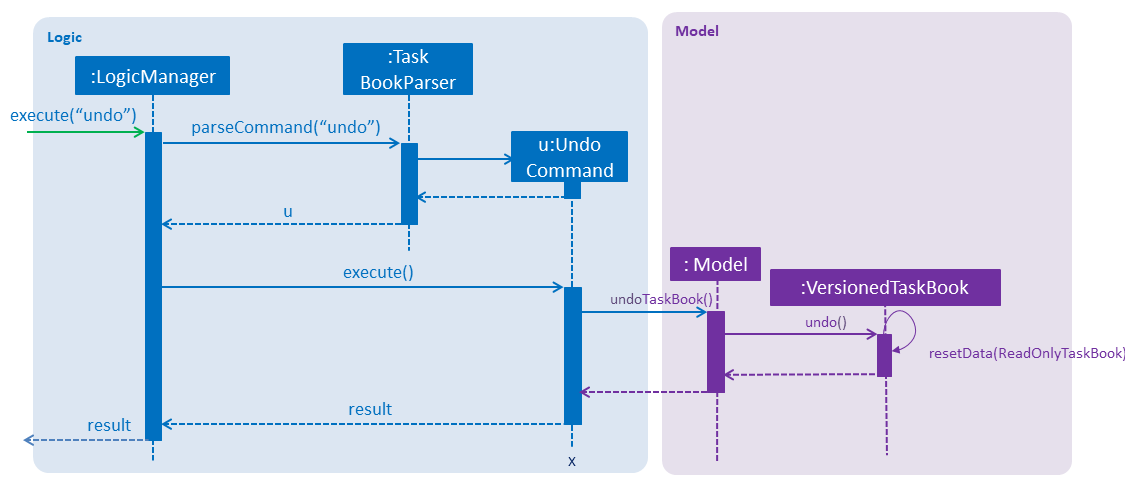
The redo
command does the opposite — it calls Model#redoAddressBook()
, which shifts the currentStatePointer
once to the right, pointing to the previously undone state, and restores the task book to that state.
If the currentStatePointer is at index taskBookStateList.size() - 1 , pointing to the latest task book state, then there are no undone task book states to restore. The redo command uses Model#canRedoAddressBook() to check if this is the case. If so, it will return an error to the user rather than attempting to perform the redo.
|
Step 5. The user then decides to execute the command list
. Commands that do not modify the task book, such as list
, will usually not call Model#commitTaskBook()
, Model#undoAddressBook()
or Model#redoAddressBook()
. Thus, the taskBookStateList
remains unchanged.
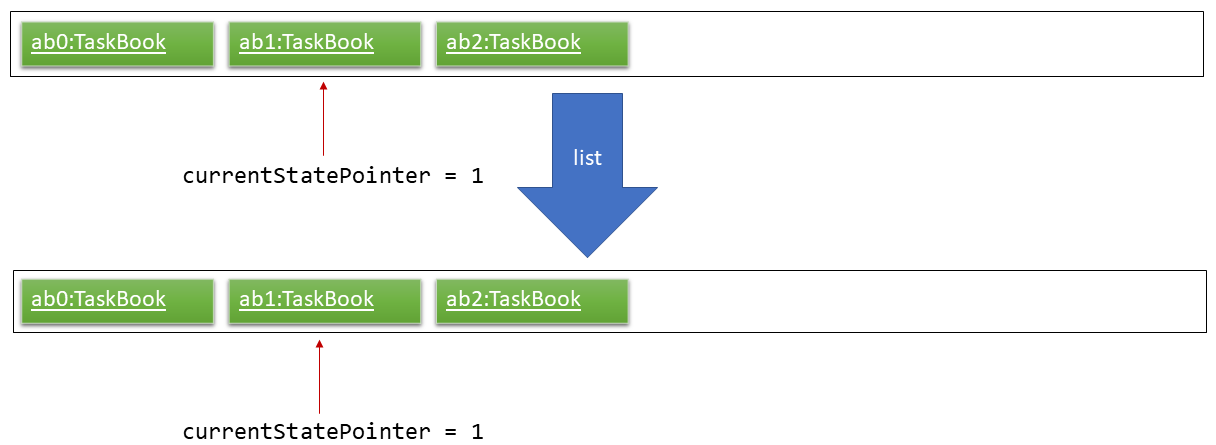
Step 6. The user executes clear
, which calls Model#commitTaskBook()
. Since the currentStatePointer
is not pointing at the end of the taskBookStateList
, all task book states after the currentStatePointer
will be purged. We designed it this way because it no longer makes sense to redo the add n/Do math homework …
command. This is the behavior that most modern desktop applications follow.
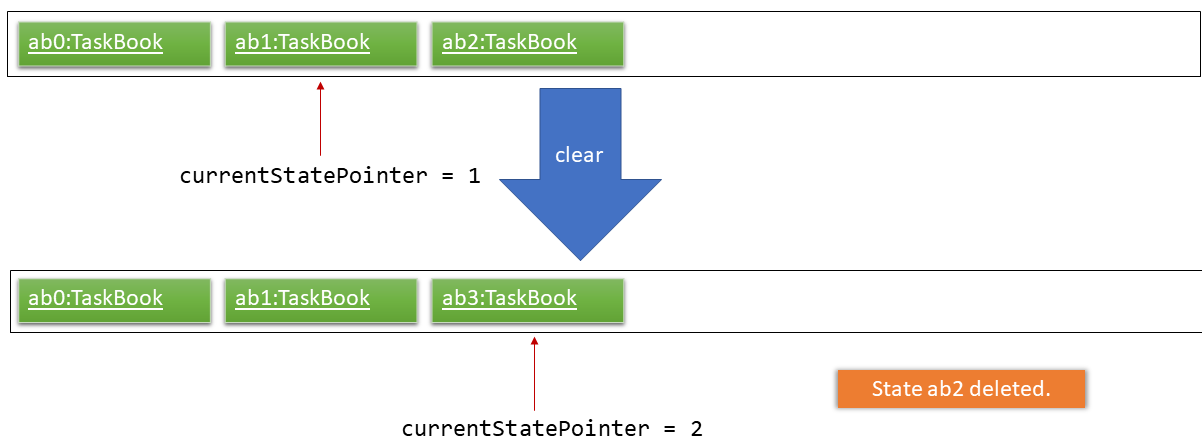
The following activity diagram summarizes what happens when a user executes a new command:
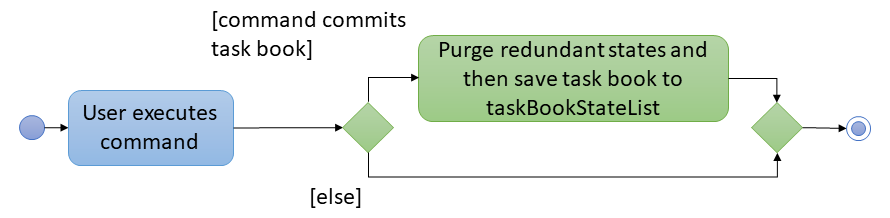
3.3.2. Design Considerations
Aspect: How undo & redo executes
-
Alternative 1 (current choice): Saves the entire task book.
-
Pros: Easy to implement.
-
Cons: May have performance issues in terms of memory usage.
-
-
Alternative 2: Individual command knows how to undo/redo by itself.
-
Pros: Will use less memory (e.g. for
delete
, just save the task being deleted). -
Cons: We must ensure that the implementation of each individual command are correct.
-
Aspect: Data structure to support the undo/redo commands
-
Alternative 1 (current choice): Use a list to store the history of task book states.
-
Pros: Easy for new Computer Science student undergraduates to understand, who are likely to be the new incoming developers of our project.
-
Cons: Logic is duplicated twice. For example, when a new command is executed, we must remember to update both
HistoryManager
andVersionedTaskBook
.
-
-
Alternative 2: Use
HistoryManager
for undo/redo-
Pros: We do not need to maintain a separate list, and just reuse what is already in the codebase.
-
Cons: Requires dealing with commands that have already been undone: We must remember to skip these commands. Violates Single Responsibility Principle and Separation of Concerns as
HistoryManager
now needs to do two different things.
-
3.4. Add Milestone feature
3.4.1. Current Implementation
Whenever a Task
object is created, it will be instantiated with an empty List
of Milestone
objects.
When the user calls the add_milestone
command,
he/she will enter an index
to select an existing Task
and the desired arguments with the appropriate prefixes. (e.g i/
INDEX m/
MILESTONE DESCRIPTION r/
RANK)
The index
represents the Task
in the list from PersonListPanel
displayed in the GUI.
The following sequence diagram illustrates how the add_milestone
command is implemented.
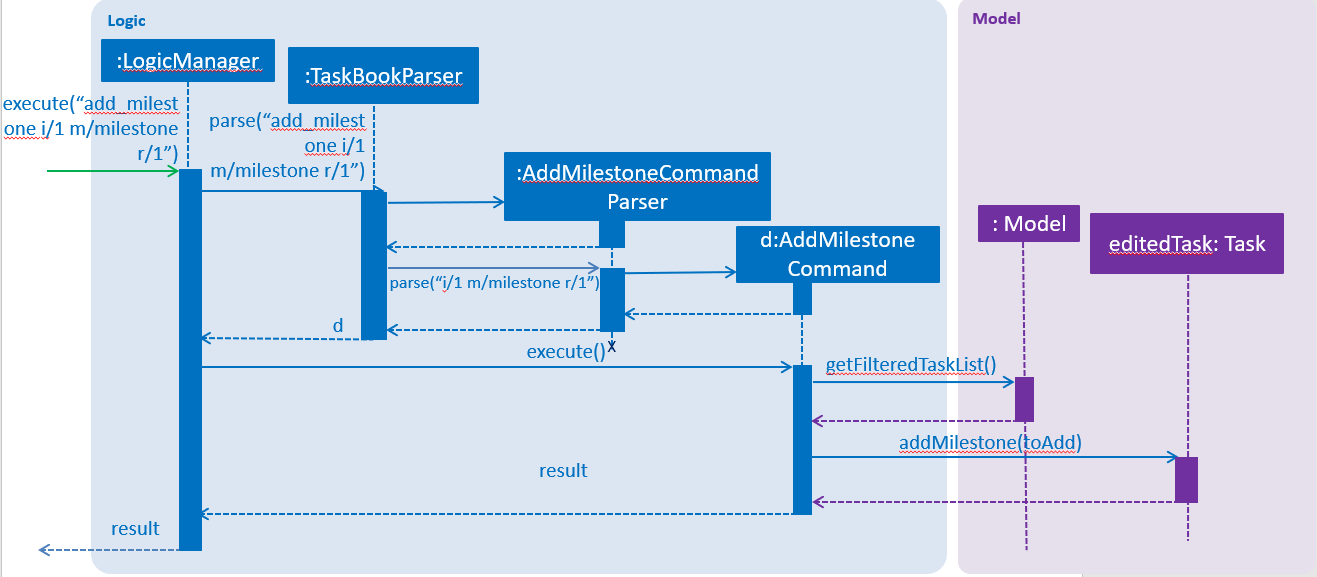
3.4.2. Design Considerations
Aspect: How to order all the milestones for each task
-
Alternative 1 (current choice): Use an
ArrayList
to implement aList
ofMilestone
objects for eachTask
andsort
them each time aMilestone
is added using a custom comparator.-
Pros: Easy to implement.
-
Cons:
List
interface does not prevent adding of duplicateMilestone
objects
-
-
Alternative 2: Use a
TreeSet
to implement aList
ofMilestone
objects for eachTask
-
Pros: Does not allow duplicate
Milestone
objects to be added. -
Cons: Requires in-depth understanding of how to use the SortedSet interface.
-
Aspect: Selecting an existing task to add a milestone to
-
Alternative 1 (current choice): Select an existing
Task
using theindex
-
Pros: Prevents ambiguity when selecting the desired
Task
using other parameters such astitle
as theindex
of eachTask
is unique and ordered -
Cons: User has to take time to scroll through the list to find the desired
Task
which can be quite troublesome and time-consuming if the list is long due to a large number of existing tasks.
-
-
Alternative 2: Select an existing
Task
using thetitle
-
Pros: More convenient for the user as there is no command to search for
index
of desiredTask
-
Cons: May result in confusion especially if there are tasks with very similar
title
.
-
3.5. Defer Deadline feature
3.5.1. Current Implementation
The defer deadline
mechanism is facilitated by VersionedTaskBook
. The defer deadline command
takes in the index
prefix
with index
of the task to be deferred, followed by the deadline prefix
with its corresponding number of days
which will defer the deadline in the task by the number of days.
The index represents the Task
in the list from PersonListPanel
displayed in the GUI
.
The validity of the input by the user is checked through the DeferDeadlineCommandParser
and TaskBookParser
. A
DeferDeadlineCommand
object is returned and the execute()
method will be performed. The validity of the index will be
checked and the task to be deferred is retrieve using the getFilterTaskList()
method. A copy of the task will be
instantiated as deferredTask. The deadline of deferredTask
will be deferred by the number of days inputted by the user.
The validity of the deadline and existence of duplicate tasks will be checked. Task to be deferred will be updated with
deferredTask
through updateTask()
and updateFiteredTaskList()
method in the Model Component.
Step 1. The user launches the application. If it is the first time he/she is launching it, the VersionedTaskBook
will be
initialized with a sample task book data. If the user has already launched it previously and made changes to it, the
VersionedTaskBook
launched will contain the data that he/she has entered in the previous launch.
Step 2. The user executes defer i/1 dd/1
to defer the deadline of the selected task at index 1 in the list. The defer
command obtains the data of the task that the user wants to change based on the input index.
Step 3. The defer
command will retrieve and copy the details of the task at index 1 and instantiate a new task
deferredTask
and defer the deadline by 1 day
.
Step 4. The defer command will call Model#updateTask()
and update the task at index 1 with the details of deferredTask
.
Also, Model#updateFilteredTaskList()
will be called to update the list that is being shown to the user with the updated task.
Step 5. Lastly, Model#commitTaskBook()
will be called causing the modified state of the task book after the defer i/1 dd/1 command
executes to be saved in the TaskBookStateList
, and the currentStatePointer
to be updated.
The following sequence diagram illustrates how the defer
command is implemented.
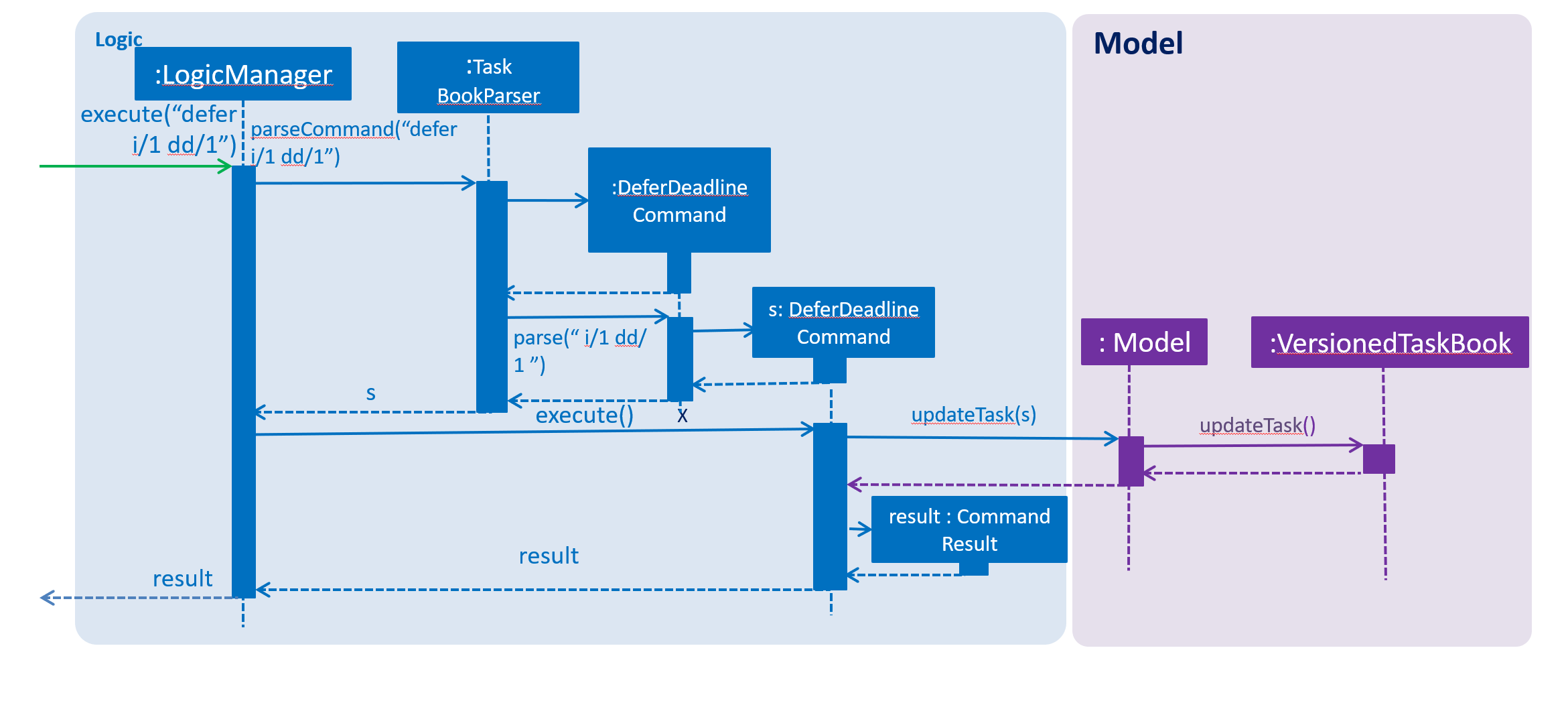
3.5.2. Design Considerations
Aspect: How should the deadline of the task be deferred
-
Alternative 1 (current choice): Choose to
defer
the task based on thenumber of days required
and the deadline will beautomatically updated
.-
Pros: More
user-friendly
as it allows user to defer the deadline by thenumber of days required
and the deadline will beautomatically updated
without the user finding the exact deadline through calendar. -
Cons: More
complex
implementation of the feature asmultiple conditional statements
are required to ensure the deadline is updated correctly by taking into consideration thefeatures of a calendar
. For example, which are the months have 30 or 31 days and how is leap year calculated?
-
-
Alternative 2: Choose to
defer
the task based on theexact date
-
Pros:
Easy
implementation of defer deadline command as just have to change the deadlinebased on users input
. -
Cons: By allowing users to
defer
the tasks by entering theexact deadline
to defer will result in defer deadline command to besimilar to edit task
as it is simply just editing the task’s deadline by keying in a new deadline.
-
3.6. Locking/Unlocking Task Book feature [coming in v2.0]
3.6.1. Proposed Implementation
To ensure encryption keys are both sufficiently random and hard to brute force,
we will use standard password-based encryption (PBE) key derivation methods.
When the student uses Task Book for the first time, he or she will be requested to enter a new password.
Since password recovery methods may not be implemented, this password must be easy to remember by the student.
Along with the password text provided by the student, a salt is appended to produce a hash (Figure below).
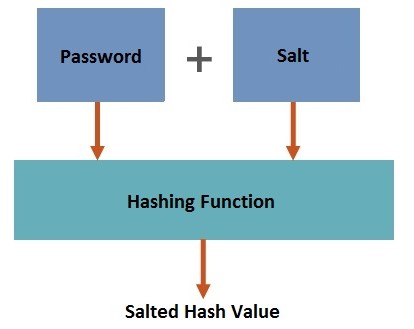
An AES or DES encryption key is thus derived from this process. To unlock the Task Book, the same salt is appended to the password provided to generate the decryption key.
In the Sequence Diagram, these are the interactions within the Logic component for the execute("lock") API call (Figure below):
-
Logic
parses the command and returns the LockCommand. -
During the command execution,
Model
checks whether a password has been set. -
If so,
Encryptor
will encrypttaskbook.xml
file so the Task Book cannot be seen by anyone without the password. -
Else, a
CommandException
is returned to prompt the user to set a password.
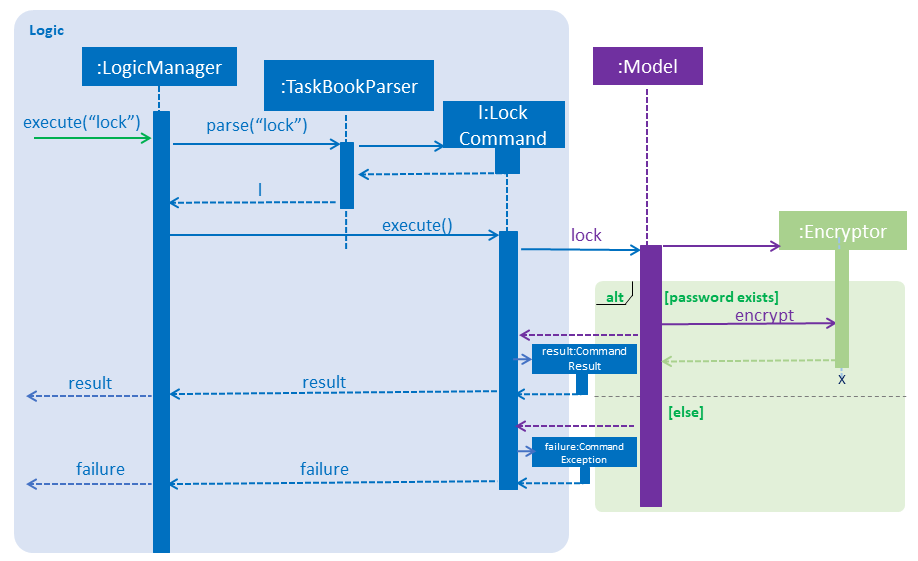
3.6.2. Design Considerations
There are a few ways to implement the password encryption for our product.
However, each method has its strengths and weaknesses. We will be explaining why we chose this particular implementation design.
Aspect: Online password authentication
-
Alternative 1: Offline password authentication
-
Pros: Simple and efficient method to log into Task Book with a lower risk of data breach
-
If student has set a complex password, it will be harder to hack into Task Book
-
-
Cons: Possible data loss if student’s password is forgotten
-
-
Alternative 2: Connect student logging session to an online authentication system
-
Pros: Allows students to reset their password, if forgotten
-
Cons: Extra step to connect to the internet and send hashed password to verify with the database. Additional space is also required in database to store users and their passwords securely.
-
4. Testing
4.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
4.2. Types of tests
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.seedu.address.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.seedu.address.storage.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.seedu.address.logic.LogicManagerTest
-
4.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
5. Dev Ops
5.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
5.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
5.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
5.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
5.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
5.6. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Product Scope
Target user profile:
-
Students who need to manage a significant number of daily tasks
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: manage daily tasks faster than Google calendar/handwritten notebook and become more productive
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
forgetful student |
add new task |
keep track of my workload |
|
efficient student |
complete a task |
keep track of my incomplete tasks |
|
indecisive student |
edits a task |
change information of my existing tasks |
|
tidy student |
delete a task |
remove tasks that I do not intend to complete |
|
organized student |
sort the tasks in the order preferred to have a more organised task list |
complete tasks with more urgent deadlines/ highest priority firsts. View tasks in a list sorted by the lexicographical order of the title or module codes. |
|
tidy student |
Add tags to tasks |
to organise, categorise and identify the tasks easily |
|
organized student |
Remove tags to tasks |
to remove the unsuitable tags that were added previously for better organisation, categorisation and identification of the tasks easily |
|
structured student |
Select a tag to view |
shows a list of tasks with the selected tag to help with the planning of work schedule for that type of tasks |
|
busy student |
defer deadlines |
allow for a more flexible schedule when workload becomes too heavy |
|
unorganised student |
select a date |
add/delete/complete tasks for that particular day |
|
objective student |
break up my task into smaller tasks |
manage them more effectively |
|
targeted student |
track the productivity of how fast tasks are being completed |
learn more about my studying habits and work more effectively |
Appendix C: Use Cases
(For all use cases below, the System is the TaskBook
and the Actor is the student
, unless specified otherwise)
Use case: Select a date
MSS
-
Student requests to select date required
-
TB checks for its validity and changes to the required date
Use case ends.
Extensions
-
1a. Date entered by the student is not valid e.g. dd/29 mm/2 yyyy/2018
-
1a1. TB prompts student to enter a correct date
Use case resumes at step 2.
-
Use case: Add new task
MSS
-
Student selects the deadline for a task
-
TB updates the selected date
-
Student requests to add a new task with some details
-
TB checks for the validity of command and adds the task to the list
Use case ends.
Extensions
-
3a. Student did not enter one or more compulsory input(s) for the task
-
3a1. TB tells student that input(s) is/are empty
Use case ends.
-
-
3b. Student enters a duplicated task
-
3b1. TB shows that task already exists in TB
Use case ends.
-
Use case: Complete task
MSS
-
Student selects the date of completed task
-
TB updates the selected date
-
Student requests to complete the task
-
TB checks for its validity and completes the task in TB
Use case ends.
Extensions
-
3a. Student attempts to complete the task in less than 1 hour
-
3a1. TB requests for student to enter a more suitable number of hour(s)
Use case resumes at step 4.
-
-
3a. Student wants to complete a completed task
-
3a1. TB gives an error to show that task is completed already
Use case ends.
-
Use case: Delete task
MSS
-
Student requests to delete a task by providing its index
-
TB removes deadline from the task
Use case ends.
Extensions
-
1a. Student provides an invalid index of the task
-
1a1. TB outputs an error message
-
Use case: Sort tasks
MSS
-
Student requests to sort his or her tasks in the lists and provides the sorting method
-
TB checks for the validity of the sorting method
-
TB displays the sorted tasks list based on the sorting method.
Use case ends.
Extensions
-
1a. Student provided an invalid sorting method
-
1a1. TB outputs an error message to request for a valid sorting method
Use case ends.
Use case: Defer deadlines
-
MSS
-
Student requests to defer the deadline for an existing task by number of days
-
TB checks the validity of the index and number of days.
-
TB updates and display the new deadline for the existing task
Use case ends.
Extensions
-
1a. Student wants to defer a deadline for an non-existent task
-
1a1. TB outputs an error message
-
-
1b. Student wants to defer to a invalid deadline for the selected task
-
1b1. TB outputs an error message
Use case ends.
-
Use case: Add tag
MSS
-
Student requests to add a new tag and provides the index and the tag
-
TB adds the tag to the selected task
Use case ends.
Extensions
-
1a. Student enters an invalid index when list of tasks added is empty
-
1a1. TB outputs error message for invalid index
Use case ends.
-
-
1b. Student enters index, tag and rank without all the required prefixes
-
1b1. TB outputs error message for invalid format
Use case ends.
-
-
1c. Student enters an invalid tag
-
1c1. TB outputs error message for invalid format
Use case ends.
-
Use case: remove tag
MSS
-
Student requests to remove a tag and provides the index and the tag
-
TB removes the tag from the selected task
Use case ends.
Extensions * 1a. Student enters an invalid index when list of tasks added is empty ** 1a1. TB outputs error message for invalid index
+ Use case ends.
-
1b. Student enters index, tag and rank without all the required prefixes
-
1b1. TB outputs error message for invalid format
Use case ends.
-
-
1c. Student enters an invalid tag
-
1c1. TB outputs error message for invalid format
Use case ends.
-
-
1d. Student selected the task that doesn’t have the tag indicated
-
1d1. TB outputs error message for invalid format
Use case ends.
-
Use case: select tag
MSS
-
Student requests to display list of task with the selected tag and provides the tag
-
TB removes the tag from the selected task
Use case ends.
-
1c. Student enters an invalid tag
-
1c1. TB outputs error message for invalid format
Use case ends. === Use case: Edit task
-
-
MSS
-
Student requests to edit a selected task by providing its index and the fields with the values to be updated.
-
TB checks for validity of the index and updates the fields with the values provided.
Use case ends.
Extensions
-
1a. Student provided an invalid index
-
1a1. TB outputs error message
-
1a2. Student enters a new Edit command
Use case ends.
-
-
1b. Student did not provide any field or values to update selected task
-
1b1. TB returns an error message
-
1b2. Student enters a new Edit command
Use case ends.
-
-
1c. Values provided by student results in the exact same task as before it was edited
-
1c1. TB returns no field edited error message
-
1c2. Student enters a new Edit command
Use case ends.
-
-
1d. Values provided by student results in an edited task exactly the same as another existing task
-
1d1. TB returns duplicate task info message
-
1d2. Student enters a new Edit command
Use case ends.
-
Use case: Add milestone
MSS
-
Student requests to add a new milestone and provides the index, milestone description and rank
-
TB adds the milestone to the selected task
Use case ends.
Extensions
-
1a. Student enters an invalid index when list of tasks added is empty
-
1a1. TB outputs error message for invalid index
Use case ends.
-
-
1b. Student enters index, milestone description and rank without all the required prefixes
-
1b1. TB outputs error message for invalid format
Use case ends.
-
-
1c. Student enters duplicate milestone description
-
1c1. TB outputs error message for duplicate milestone description
Use case ends.
-
-
1d. Student enters duplicate rank
-
1d1. TB outputs error message for duplicate rank
Use case ends.
-
Appendix D: Non Functional Requirements
Here are some conditions that are not explicitly stated in the features that Task Book provides, but are crucial features that allow users to operate the system functionally.
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should be able to respond within 2 seconds.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
-
Will be offered free for students.
-
Not built to contain sensitive information due to lack of password protection.
-
Tasks dated as far as 10 years ago may be difficult to retrieve, unless data is backed up in the cloud storage.
Appendix F: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
F.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
{ more test cases … }
F.2. Selecting a date as a deadline
-
Select a deadline
-
Test case:
select 1
Expected: No deadline selected. Message of invalid command format error is shown. -
Test case:
select dd/1
Expected: No deadline selected. Message of invalid command format error is shown. -
Prerequisite: Select command has not been called before.
Test case:select 1/1
Expected: Deadline of 1/1/2018 is selected. Message of select success is shown. -
Prerequisite: Select command has not been called before.
Test case:select dd/1 mm/1
Expected: Deadline of 1/1/2018 is selected. Message of select success is shown. -
Prerequisite: Latest select command selected a deadline with 2020 as year (e.g.1/1/2020)
Test case: 'select 1/1`
Expected: Deadline of 1/1/2020 is selected. Message of select success is shown. -
Prerequisite: Latest select command selected a deadline with 2020 as year (e.g.1/1/2020)
Test case: 'select dd/1 mm/1`
Expected: Deadline of 1/1/2020 is selected. Message of select success is shown. -
Test case:
select 1/1/2018
Expected: Deadline of 1/1/2018 is selected. Message of select success is shown. -
Test case:
select dd/1 mm/1 yyyy/2018
Expected: Deadline of 1/1/2018 is selected. Message of select success is shown. -
Test case:
select 29/2/2018
Expected: No deadline selected. Message of invalid date error is shown. -
Test case:
select 1/13/2018
Expected: No deadline selected. Message of invalid date error is shown. -
Test case:
select 1/1/201
Expected: No deadline selected. Message of invalid date error is shown. -
Test case:
select a29/2/2018
Expected: No deadline selected. Message of deadline contains illegal characters error is shown. -
Test case:
select 01/01/02018
Expected: Deadline of 1/1/2018 is selected. Message of select success is shown.
-
-
Updated filteredTaskList shown after selecting deadline
-
Prerequisites: Multiple tasks in the list with different deadlines. At least one task has the deadline of 1/1/2018.
-
Test case:
select 1/1/2018
Expected: Deadline of 1/1/2018 is selected. Filtered task list only shows tasks with deadline of 1/1/2018. -
Test case:
select 29/2/2018
Expected: No deadline selected. Filtered task list will not be updated and will show what is was showing previously.
-
F.3. Deleting a task
-
Deleting a task while all tasks are listed
-
Prerequisites: List all tasks using the
list
command. Multiple tasks in the list. -
Test case:
delete 1
Expected: First contact is deleted from the list. Details of the deleted contact shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No task is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size) {give more}
Expected: Similar to previous.
-
{ more test cases … }
F.4. Editing a task
-
Editing a task while all tasks are listed
-
Prerequisites: List all tasks using
list
command. Only 3 tasks are in the list. -
Test case:
edit
Expected: Task is not edited. Message of invalid command format is shown. -
Test case:
edit 1
Expected: Task is not edited. Message of invalid command format is shown. -
Test case:
edit t/Do CS2113 tutorial
Expected: Task is not edited. Message of invalid command format is shown. -
Test case:
edit i/
Expected: Task is not edited. Message of empty index is shown. -
Test case:
edit i/1 t/
Expected: Task is not edited. Message of empty title is shown. -
Test case:
edit i/1 d/
Expected: Task is not edited. Message of empty description is shown. -
Test case:
edit i/1 c/
Expected: Task is not edited. Message of empty module code is shown. -
Test case:
edit i/1 p/
Expected: Task is not edited. Message of empty priority level is shown. -
Test case:
edit i/1 h/
Expected: Task is not edited. Message of empty hours is shown. -
Test case:
edit i/0 t/Do CS2113 tutorial
Expected: Task is not edited. Message of invalid index (index must be a non-zero unsigned integer) is shown. -
Test case:
edit i/4 t/Do CS2113 tutorial
Expected: Task is not edited. Message of invalid task displayed index (the task index provided is invalid) is shown. -
Prerequisites: Tasks in list do not have the exact same fields.
Test case:edit i/1 t/Do CS2113 tutorial
Expected: Task at index 1 is edited and the original title is replaced by "Do CS2113 tutorial". Message of edit success is shown. -
Prerequisites: Task at i/1 is exactly the same another existing task, existingTask, except for the title. The title of existingTask is "Do CS2113 tutorial".
Test case:edit i/1 t/Do CS2113 tutorial
Expected: Task is not edited. Message of duplicate task error is shown. -
Prerequisites: Task at i/1 has a title of "Do CS2113 tutorial".
Test case:edit i/1 t/Do CS2113 tutorial
Expected: Task is not edited. Message of task not edited error is shown.
-
F.5. Adding a task
-
Select a particular date
-
Select the date using the
select
command, or the date-picker in the UI. -
Test case:
select 11/11/2018
Expected: Tasks with the similar deadline are listed. Timestamp in the status bar is updated.
-
-
Add a task with title, description, priority and expected number of hours entered.
-
Test case:
add t/Do project portfolio d/convert to pdf format p/high h/3 c/CS2101
Expected: A task will be added to the list, together with the other tasks with the same deadline. -
Other incorrect add commands:
add
oradd t/ d/ p/ h/
with compulsory fields not entered oradd t/Do coding d/very fun p/midhigh h/1
with invalid priority level.
-
F.6. Saving data
-
Dealing with missing/corrupted data files
-
If the file is corrupted due to illegal values in the data
-
Go to
data/taskbook.xml
and delete the file
-
-
If the file is missing:
-
The filename may be incorrect, i.e. not
taskbook.xml
, or -
taskbook.xml
may not be in the/data
folder
-
-